[vscode] - code doesn't work in vscode, but does in Blender's text editor.
When using JacquesLucke's blender_vscode extension for VScode, the following code doesn't work.
But if you use this code in Blender's native text editor, it works.
Any ideas what I'm missing?
import bpy
class DRAGGABLEPROP_OT_subscribe(bpy.types.Operator):
bl_idname = "draggableprop.subscribe"
bl_label = ""
stop: bpy.props.BoolProperty() # This is used so we don't end up in an infinite loop because we blocked the release event
def modal(self, context, event):
if self.stop:
context.scene.my_prop.is_dragging = False
print("End Dragging !")
return {'FINISHED'}
if event.value == 'RELEASE': # Stop the modal on next frame. Don't block the event since we want to exit the field dragging
self.stop = True
return {'PASS_THROUGH'}
def invoke(self, context, event):
self.stop = False
context.window_manager.modal_handler_add(self)
return {'RUNNING_MODAL'}
class TEST_PT_draggable_prop(bpy.types.Panel):
bl_label = "Test my draggable prop"
bl_space_type = 'PROPERTIES'
bl_region_type = 'WINDOW'
bl_context = "scene"
def draw(self, context):
layout = self.layout
layout.prop(context.scene.my_prop, "value")
layout.prop(context.scene.my_prop, "is_dragging")
def update_prop(self, value):
if self.is_dragging:
print("Dragging the slider !")
else:
print("Beginning dragging the slider !")
self.is_dragging = True
bpy.ops.draggableprop.subscribe('INVOKE_DEFAULT')
class DraggableProp(bpy.types.PropertyGroup):
value: bpy.props.IntProperty(update=update_prop, min=0, max=100)
is_dragging: bpy.props.BoolProperty()
def register():
bpy.utils.register_class(DRAGGABLEPROP_OT_subscribe) # Register modal operator
bpy.utils.register_class(TEST_PT_draggable_prop) # Register Panel to see what's going on
bpy.utils.register_class(DraggableProp) # Register our custom prop
bpy.types.Scene.my_prop = bpy.props.PointerProperty(type=DraggableProp) # Setup our custom prop
def unregister():
bpy.utils.unregister_class(DRAGGABLEPROP_OT_subscribe)
bpy.utils.unregister_class(TEST_PT_draggable_prop)
bpy.utils.unregister_class(DraggableProp)
del bpy.types.Scene.my_prop
if __name__ == "__main__":
register()
Comments
What's the error?
Have you watched this?
There is none, it seems to run, but nothing shows up.
I have, it's a good one!
@theoryshaw I think the problem is in how the extension is running the file. I suspected that register() is never actually executed, so I added the following code:
The resulting output was:
name is : <run_path>
Compare this to the output when running in blender:
name is : main
this should print if name == 'main'
The solution? Remove the
if __name__ == "__main__":
and just call register() when running from vscode.@theoryshaw for further info: https://docs.python.org/3/library/runpy.html
nice!...
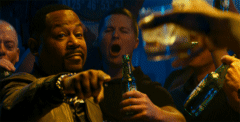