Hi, i'm looking for a Blender way to model surfaces based on survey points. Points come from CloudCompare after saving to .ply. The [F] fill option does not work that well. I read about some potential (old) addons.
This is called Delaunay Triangulation. Here's a script I roughly adapted from a ChatGPT answer. I used it on a project last month. You'll need to install scipy.
import bpy
import bmesh
from scipy.spatial import Delaunay
import numpy as np
def delaunay():
# Check if there is an active object and it's a mesh
if bpy.context.active_object is not None and bpy.context.active_object.type == "MESH":
# Get the active object
obj = bpy.context.active_object
# Get vertices from the currently selected mesh
vertices = np.array([v.co for v in obj.data.vertices])
# Create a new mesh and a new object
mesh = bpy.data.meshes.new(name="DelaunayMesh")
delaunay_obj = bpy.data.objects.new("DelaunayObj", mesh)
# Add the object to the scene
bpy.context.collection.objects.link(delaunay_obj)
# Get a BMesh representation
bm = bmesh.new()
# Add vertices to BMesh
for v in vertices:
bm.verts.new(v)
# Ensure the vertices table is populated
bm.verts.ensure_lookup_table()
tri = Delaunay([v[:2] for v in vertices]) # Use only x, y for triangulation
# Create faces based on Delaunay triangulation
for simplex in tri.simplices:
bm.faces.new([bm.verts[i] for i in simplex])
bm.to_mesh(mesh)
bm.free()
# Optional: Deselect the original object and select the new Delaunay mesh object
bpy.ops.object.select_all(action="DESELECT")
delaunay_obj.select_set(True)
bpy.context.view_layer.objects.active = delaunay_obj
else:
print("No active mesh object selected.")
if __name__ == "__main__":
delaunay()
That works very nice. Thanks for this useful tip!
With quadremesh and saved as IFC. We are able to model plots nicely with dutch landregistry data (based on AHN).
The Delauny.py code does end with some error on my site. Regards
Comments
This is called Delaunay Triangulation. Here's a script I roughly adapted from a ChatGPT answer. I used it on a project last month. You'll need to install scipy.
That works very nice. Thanks for this useful tip!
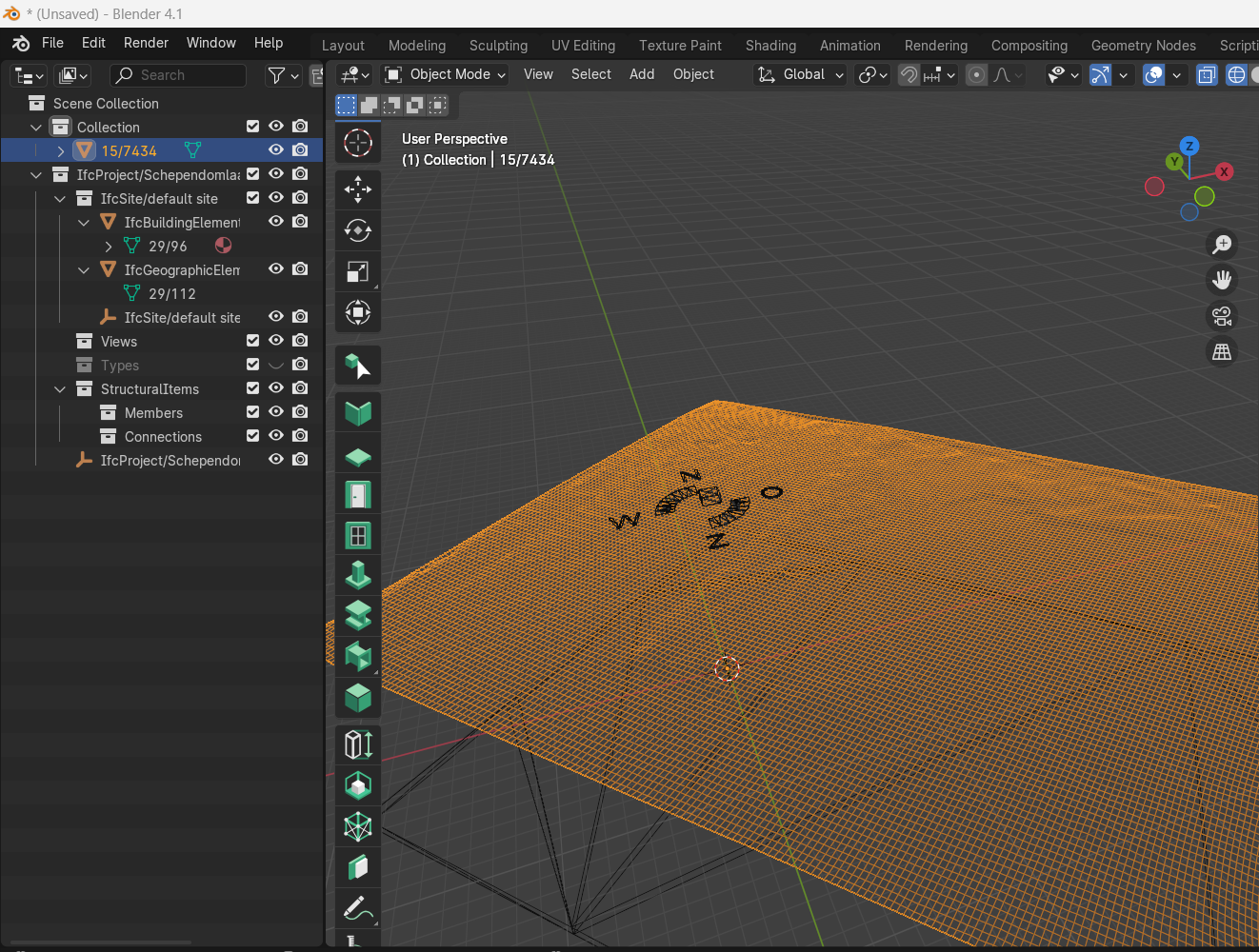
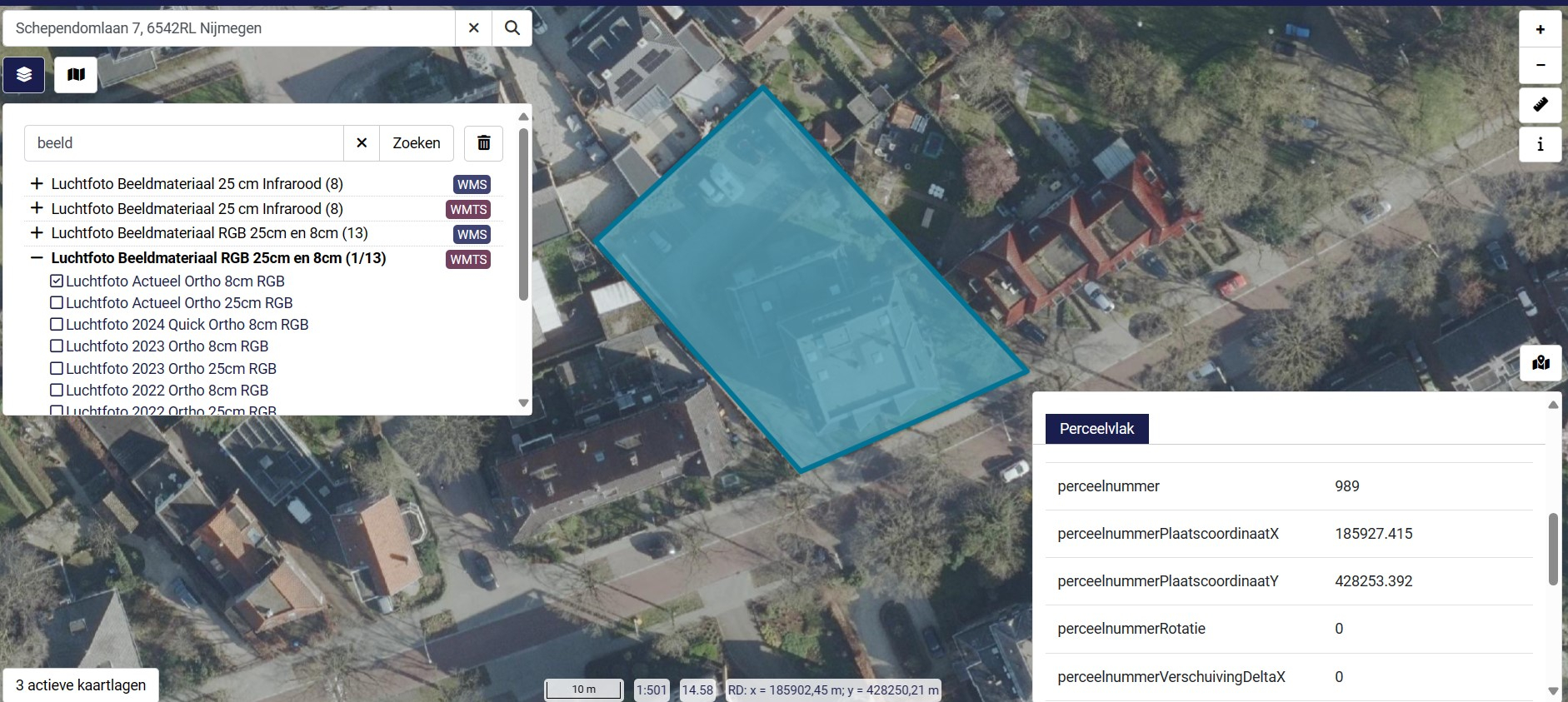
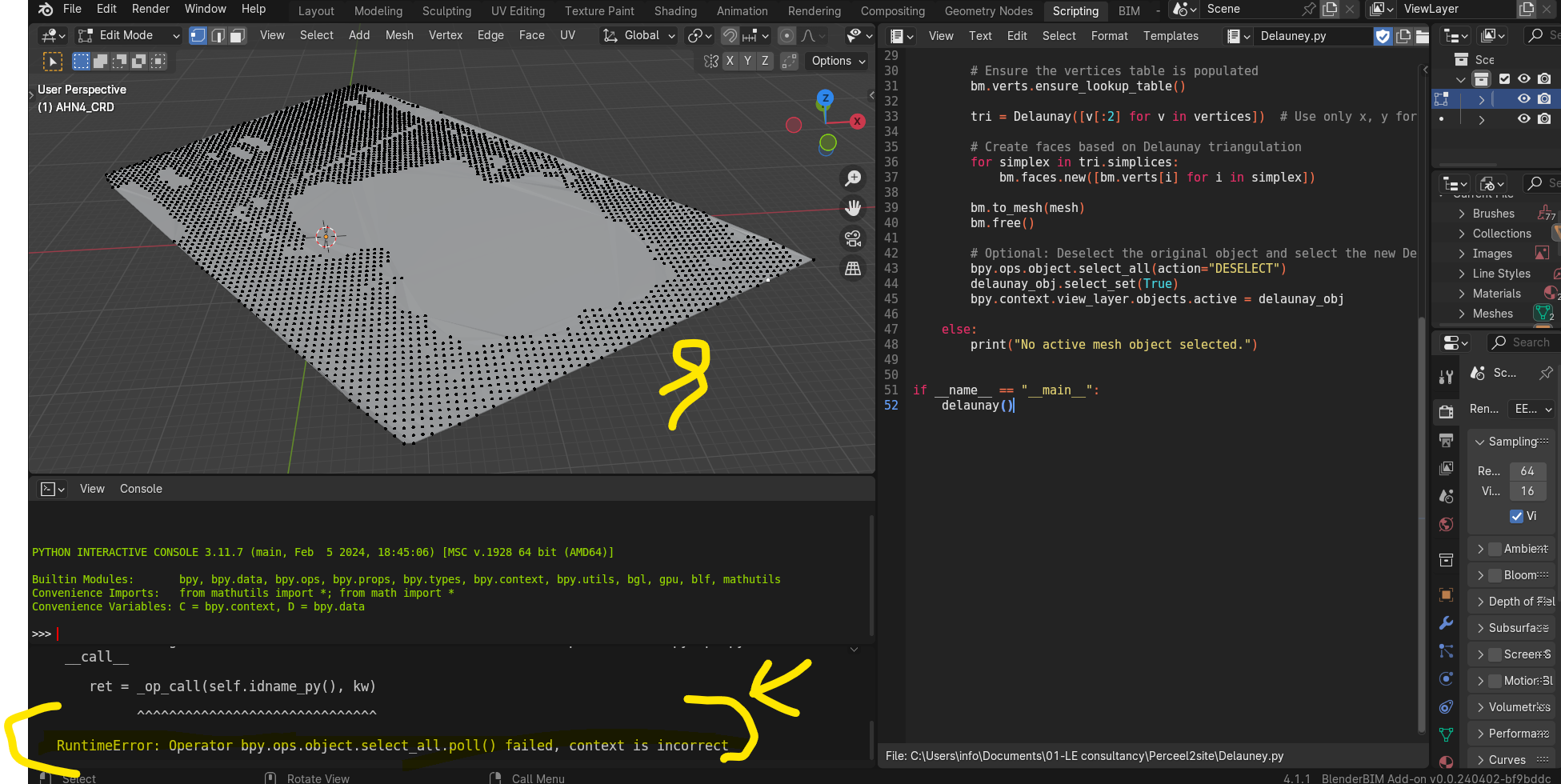
With quadremesh and saved as IFC. We are able to model plots nicely with dutch landregistry data (based on AHN).
The Delauny.py code does end with some error on my site. Regards
Yeah you can remove the 3 lines just after the # Optional comment line, they are superfluous and I think they're the culprit ones