Blender: brick wall code in Python
This is not really related to BlenderBIM but more to Blender, I was bored this friday and decided to try and learn the Blender python API.
I wanted to start of small by just creating a brick, after I created a brick. I tried creating a brick wall. This was the result. There could be a lot of improvement in the code. Using while loops etc. And probably there are existing add-ons I'm not aware off. But they all seem to be brick wall add-ons for other purposes.
But I thought it would be funny to share this here so maybe I can learn something from others. :-)
Here is the code:
import bpy
import mathutils
from itertools import repeat
mesh_name = "brick"
collection_name = "Collection"
def make_brick_wall(bricks_y_direction, bricks_z_direction, bed_joint, horizontal_joint):
#hardcode coordinates of vertices of brick
vertices_brick = [ (0,0,0),
(0,1,0),
(2.1,1,0),
(2.1,0,0),
(0,0,0.5),
(0,1,0.5),
(2.1,1,0.5),
(2.1,0,0.5)
]
edges = []
faces = [(0,1,2,3),
(4,5,6,7),
(0,4,5,1),
(1,5,6,2),
(2,6,7,3),
(3,7,4,0)
]
new_mesh = bpy.data.meshes.new('brick_mesh')
new_mesh.from_pydata(vertices_brick, edges, faces)
new_mesh.update()
# make object from mesh
new_object = bpy.data.objects.new(mesh_name, new_mesh)
# make collection
new_collection = bpy.data.collections.new(collection_name)
# add object to scene collection
new_collection.objects.link(new_object)
x = 0.0
y = 0.0
z = 0.0
x_vector = (vertices_brick[2][0])/2 #=1.1
z_vector = (vertices_brick[4][2])+bed_joint#=0.6
x_move_list=[]
xyz_list = []
x_move_list.extend(repeat(x_vector,bricks_z_direction))
for b in range (0,len(x_move_list)):
x_move_list.insert(b*2,0)
z_list = [x * z_vector for x in range(0, len(x_move_list))]
#amount of brick in x-direction
for i in range(0,bricks_y_direction):
x += (vertices_brick[2][0]) + horizontal_joint
for x_move, z in zip(x_move_list, z_list):
xyz_list.append([x+x_move, 0.0, z])
add_row(object=new_object, x=x+x_move, y=y, z=z)
def add_row(object, x, y, z):
C = bpy.context
new_object = object
new_obj = new_object.copy()
new_obj.animation_data_clear()
C.collection.objects.link(new_obj)
# one blender unit in x-direction
vec = mathutils.Vector((x, y, z))
inv = new_obj.matrix_world.copy()
inv.invert()
# vector aligned to local axis in Blender 2.8+
vec_rot = vec @ inv
new_obj.location = new_obj.location + vec_rot
make_brick_wall(bricks_y_direction=15,
bricks_z_direction=15,
bed_joint=0.1,
horizontal_joint=0.1)
And ofcourse I tried exporting my brick wall with the BlenderBIM add-on to IFC
Comments
Nice :-) it's helpful for beginners like me. Just a curiosity, why bricks have (2.1, 1, 0.5) meter lenght?
@Massimo
I was still developing, I have refactored the script a bit. Now it's possible to give in dimensions of the brick in a more understandable way.
So, i was curious about the strange brick dimensions (from what i knew) and i found that bricks have different dimensions in different countries.
Seems that there are mainly two types of standard (at least in Europe):
I thought Italy dimensions were a ISO standard, but i was wrong.
Yeah coen used the most common Dutch dimensions, 210x100x50mm (waalformaat). But there are a lot of different sizes, especially if you're renovating an old building. Then the stones can be different depending on the region. See: https://nl.m.wikipedia.org/wiki/Lijst_van_baksteenformaten
Yesterday I visited Pisa (Italy) and noticed that bricks used by arch are sometimes even 400x100x400 or more. Woah!
If you are interested in bricks and patterns than I can recommend this book 'Het Zinderend oppervlak' https://www.naibooksellers.nl/het-zinderend-oppervlak-metselwerkverband-als-patroonkunst-en-compositiegereedschap-koen-mulder.html For flip trough see the movie.
It's written in dutch but very graphical.
Hi,
While interresting at first glance, my own tests (in archipack) with "real" brick based geometry in blender's context show that blender provide a pretty limited support for complex object(s), making such approach somehow pointless.
1 building bricks as objects lead to database overflow pretty quickly (~20k bricks are enough to slow down everything).
2 bricks as single object maybe too complex for boolean to handle in timely fashion - even fast solver will be slow with such geometry, and likely to fail with coplanar geometry wich may often occurs. Checking opening shape and adjusting neighboor bricks according on python side is not a realistic way to go.
From code point of view, would rely on bmesh module in order to build a single object, as it is way faster than everything else. Probably filling vertex + faces + optional uvs and material indexed array and then feed a bmesh with data.
@stephen_l
Thank you for the insightful comment. I learn something new everday. :-)
This weekend I was trying to program a rowlock for learning purposes,
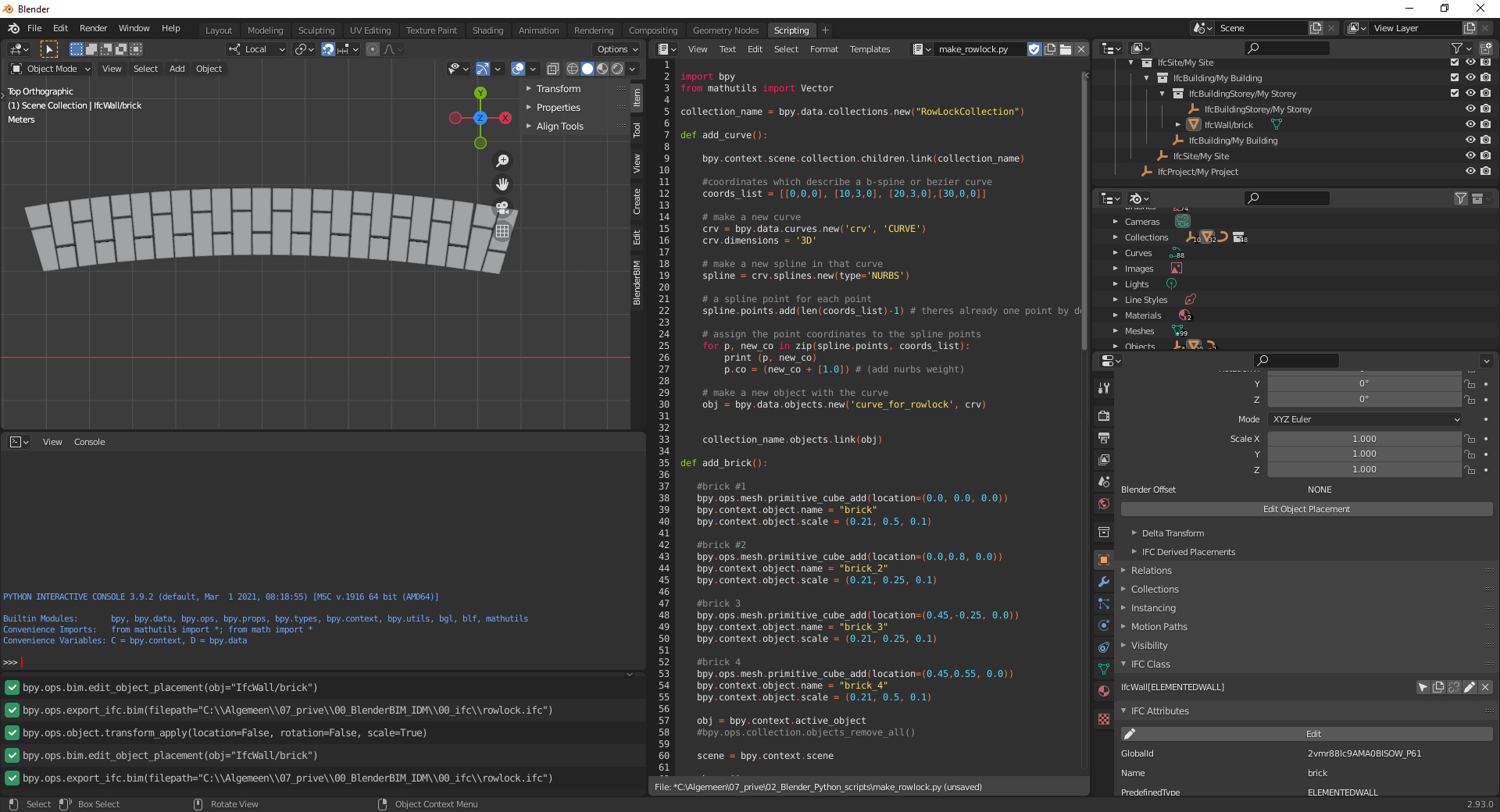
I used a NURBS curve, then an array and curve modifier to array the meshes along the curve with python, this was the result in Blender.
I converted everything to a mesh before exporting to IFC.
This was the result in IFC, it seems the mesh did not scale correctly? What am I doing wrong? Or might there be an existing or another solution on quickly making a brick rowlock in Blender? Also the curve modifier seems to warp my brick meshes at the end a bit too much.
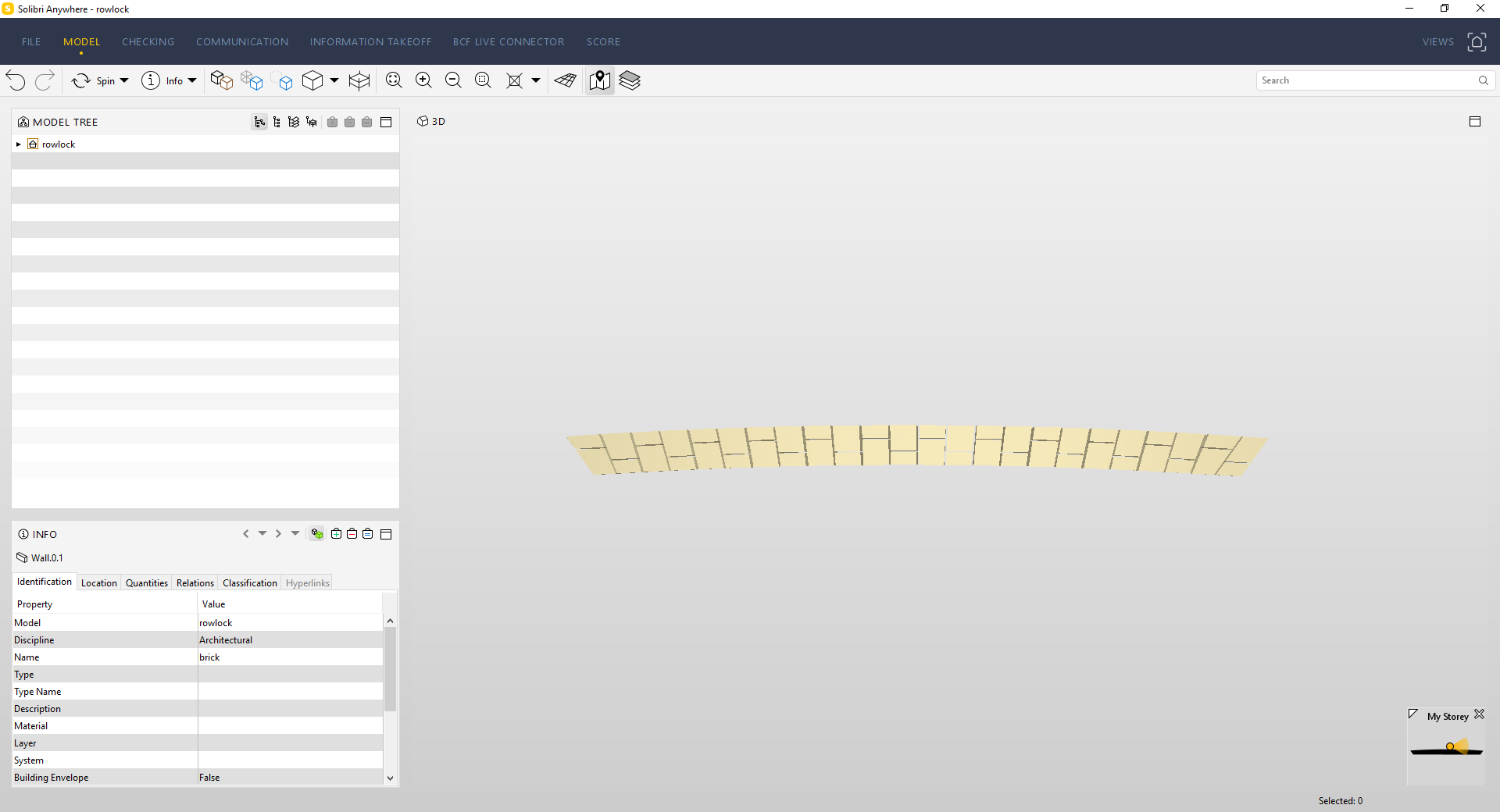
Try applying all scale modifiers. In the world of BIM, unfortunately, scaling is something avoided.
@Moult
I set the scale of the object with before exporting to IFC
It worked, the scale is set correctly now in IFC.
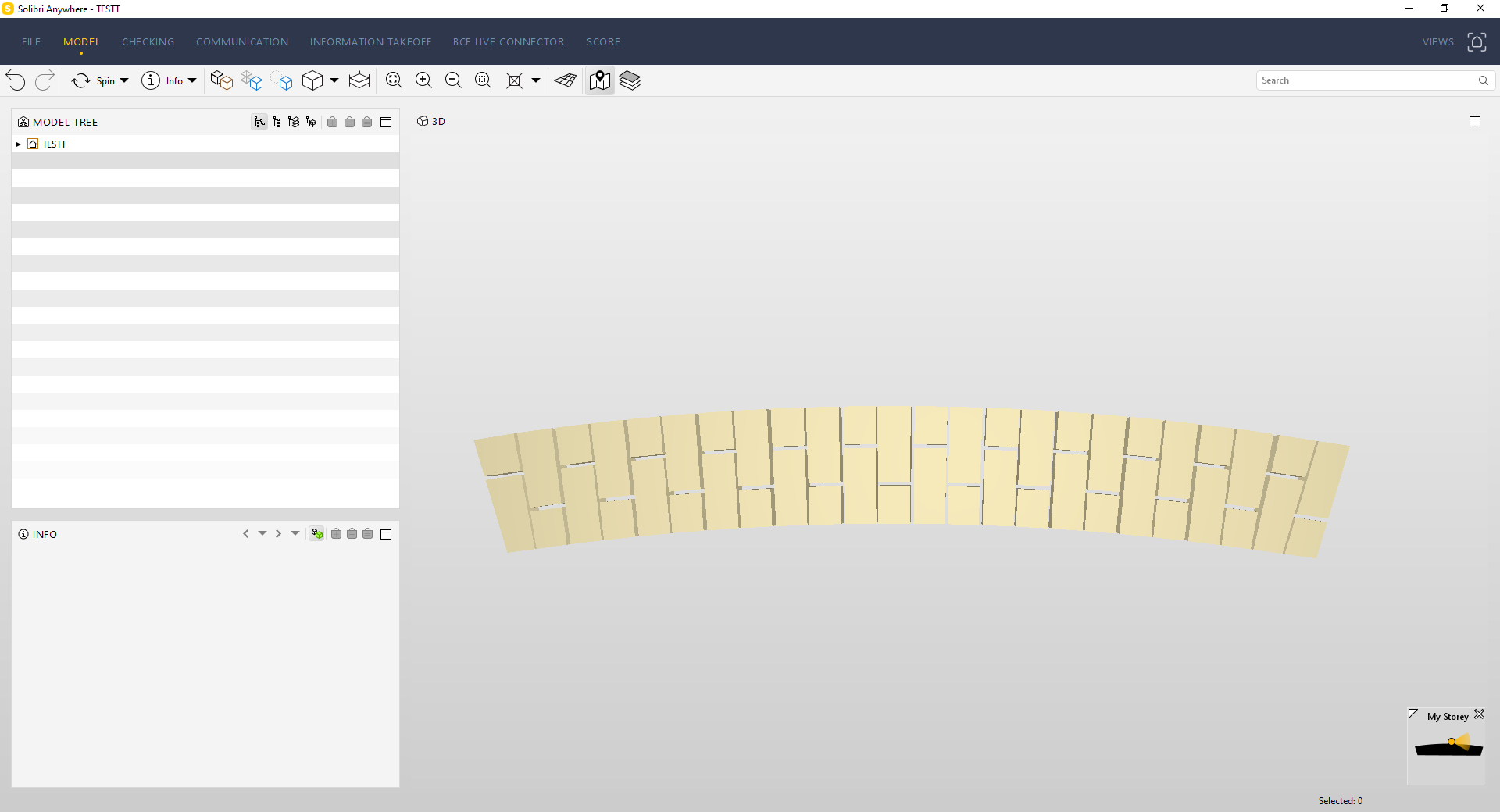
Script for the rowlock is here, but it's not very elegant yet.
Are there any existing Blender add-ons for brick detailing?
There is the Wall Factory that you can take inspiration from :
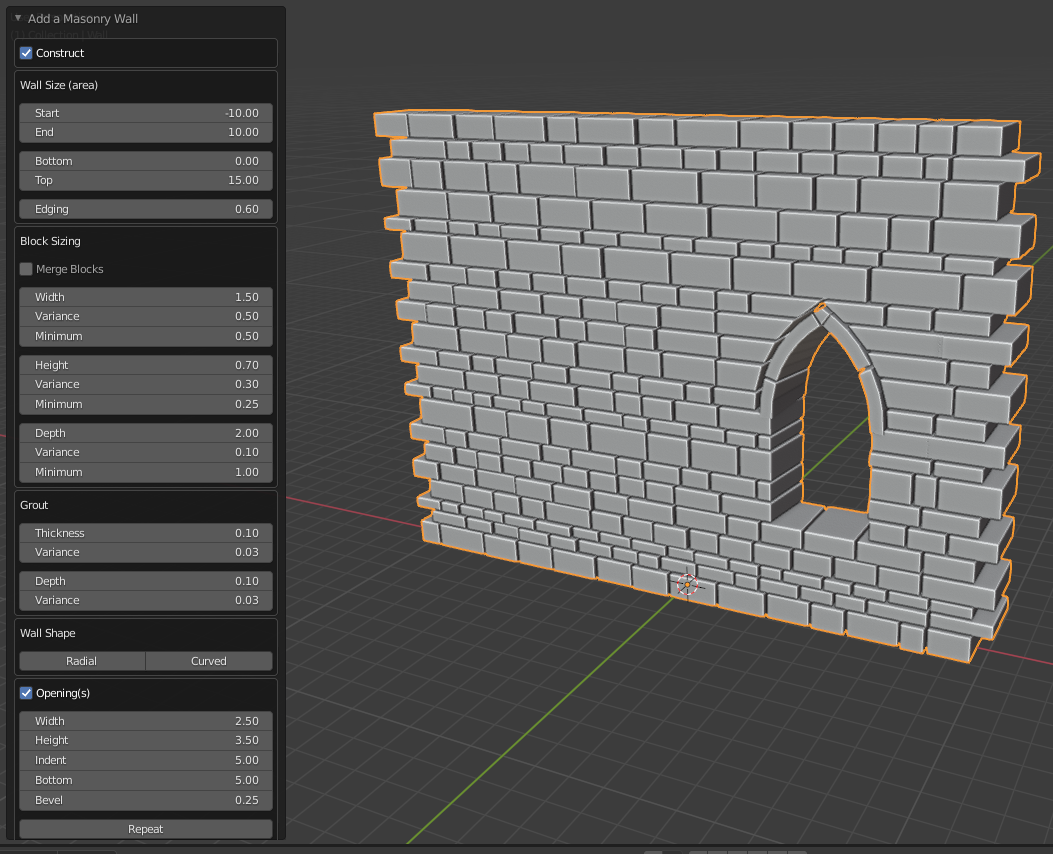
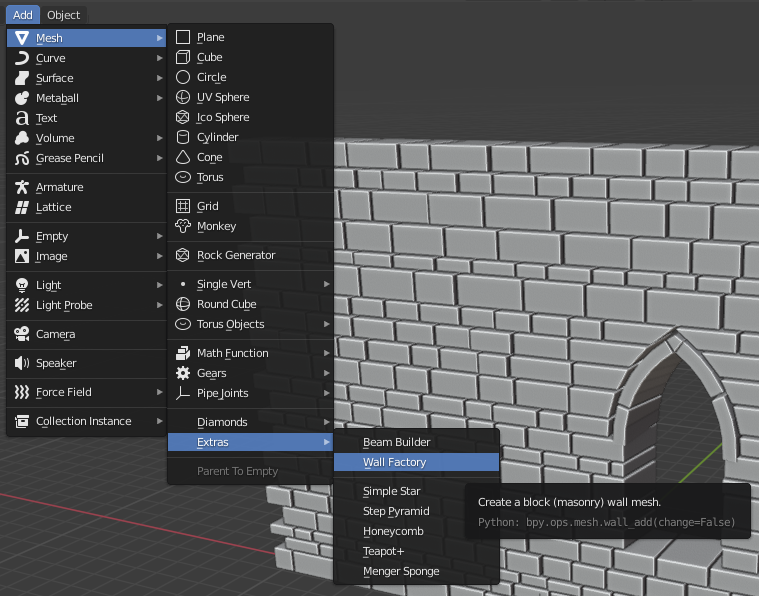
You need to enable the Add Mesh : Extra Objects add-on that's shipped with Blender.
Then go into Add > Mesh > Extras > Wall Factory
@Gorgious
Thanks for the post on how to make a blender add-on.
For the moment I was still hobbying with my brick wall script. I did a lot of refactoring, and some complete rewriting of code.
Here is the python code to create a brick wall at the moment, with different brick sizes, bed joint sizes, header joint sizes, wall length and wall height.
It only works with stretcher bond patterns at the moment, my plan is to add the possibility to add more bond patterns.
Here is the result of playing with the script, created some parametric walls within seconds. And used the Blender BIM add-on to export to IFC.
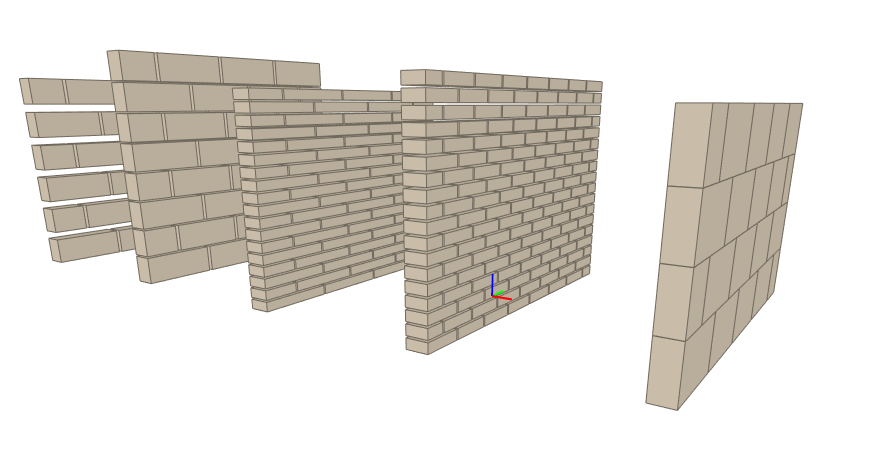
For the moment I place the mesh outside the collection, a bit of a silly method. I will keep working on user friendliness and functionality.
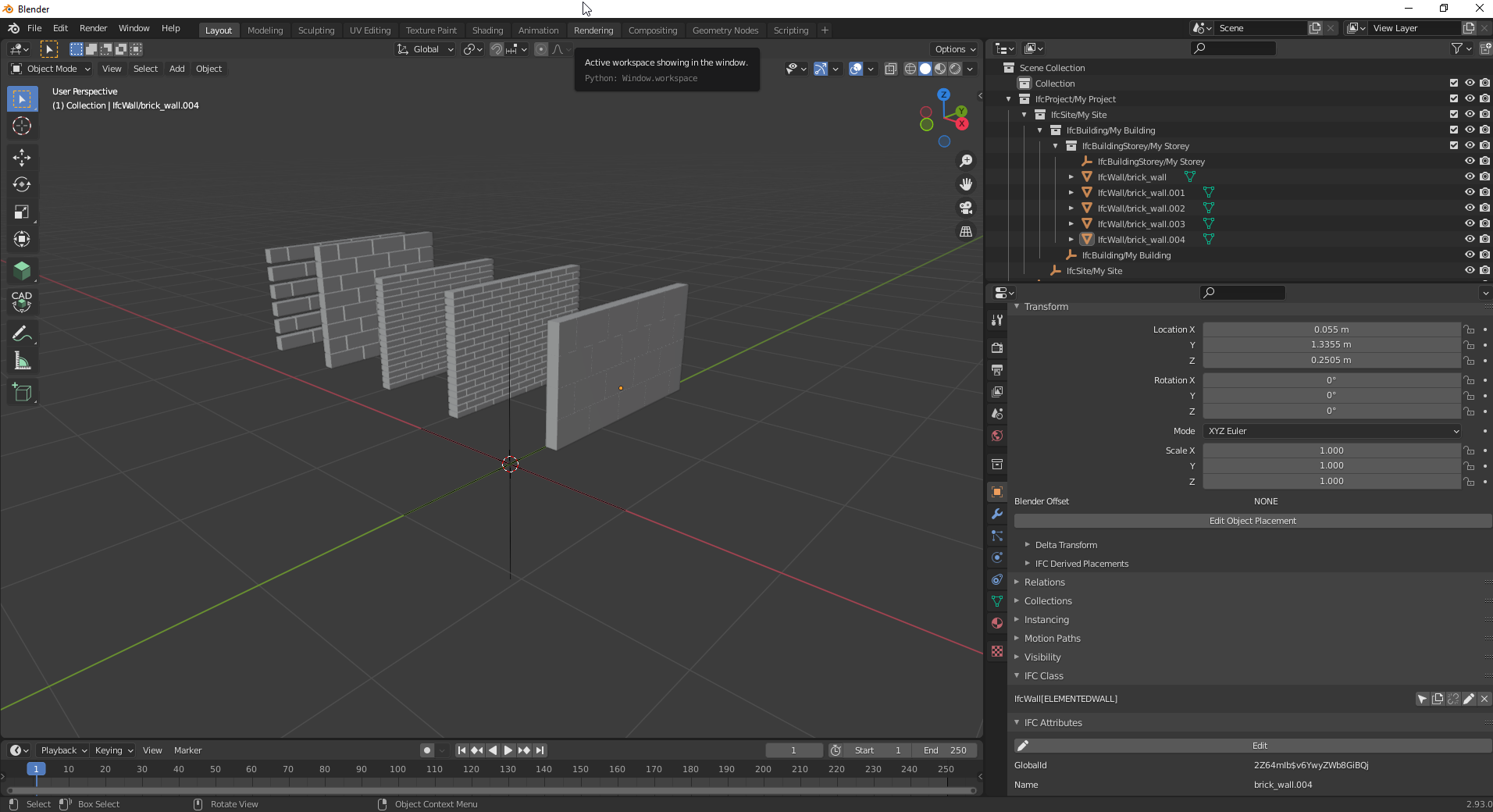
You are absolutely right about everything you said, but it didn't prevent me from trying because I am stubborn and was curious what would happen.
The bricks now includes mortar. Then I tried cutting an opening/boolean. Just like you said, it took for ages, haha.
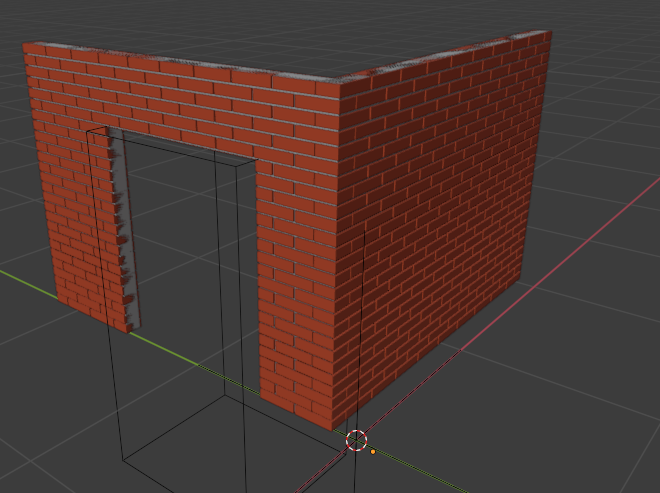
IFC results are shown in BimCollabZoom and BIMVision, for some reason, BIMVision won't load the mortar IFC object.
Ifc file is attached to this post.
I recently learned IFC4 has texture support, how would I add a brick texture to an IfcWall?
Can BlenderBIM do it?
Hi @Coen I am on my phone and am pretty useless using it, so I am just pasting the thread name... Understanding how textures and shaders work in IFC
Dion explains how textures and materials work, and how I am not sure if that is what you are looking for?
As I understand it's definitely possible to define textures in the IFC4 schema, now the problem is how the different softwares interpret this data and implement displaying these textures
@Nigel and @Gorgious
I remember seeing a LinkedIn post where someone shared a textured IFC of a log. ( A literal tree log).
I tried to import the IFC in BlenderBIM, but BlenderBIM crashed each time.
Can't find this LinkedIn post now, maybe someone knows what I'm talking about.
@Coen Do you mean this post?
https://www.linkedin.com/posts/jan-brouwer-009b5229_sketchup-activity-6901282805045624832-mZ2B
@Massimo
Exactly what I was looking for, thank you!
I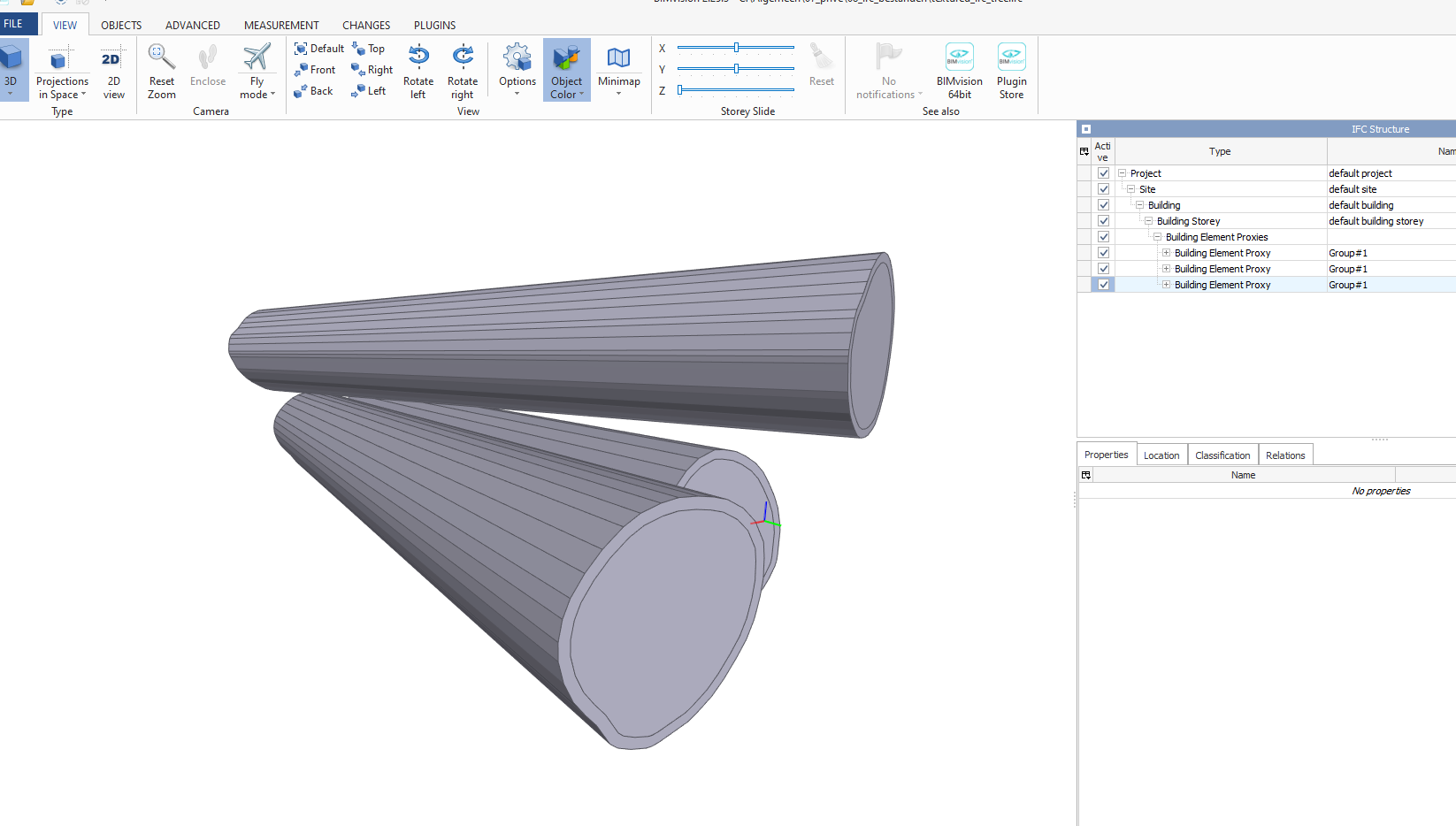
IFC Loads in BIMVision without textures.
Blender crashes when trying to import this IFC, just curious if it's just me...
Also just saw this on LinkedIn
https://80.lv/articles/a-new-geometry-nodes-based-brick-wall-generator-for-blender/