Don't forget to cast the value since you're using a string.
I have no idea what you mean by this ?
I mean you're using a StringProperty to hold the value of my_length. It's representing a numerical value so I assume you have a reason to use a String Property instead of a FloatProperty. There are valid reasons to do so, mainly because float values are limited in precision, as you can see in your gif when your change a value the last digits of the length kind of go haywire because of that. Strings can hold an infinite precision (at least in the needed significance range for construction projects).
Internally it is stored as a string of characters, not as a numerical value. Python is a dynamic language which lets you do a lot of things without worrying too much about the underlying data structure, but you have to be careful about some things. print("2") and print(2) will yield the same result, however print("2" + 2) will raise an error. The interpreter can't assume how you want to compute non-trivial operations. So I'd recommend you to cast the value to the intended data structure when you're dealing with dynamic data types. print("2" + str(2)) will yield "22" when print(float("2") + 2) will yield "4.0"
Thanks for the help, I am trying to make some experimental add-on in which all the geometry is completed IFC based. Don't have any use case but I'm just exploring possibilities of what is possible and could be practical.
I hope this is a very simple question, but gooling gave me unsatisfying results:
I have the following add-on :
The workflow should be as following:
0) folder icon sets a path
1) > icon adds an image in Blender, so you could make transformations to the image in Blender, e.g. scaling, rotation and position
2) + icon saves the path images with transformation as an IfcPropertySet under IfcProject
3) Load image(s) from IFC loads the images with transformations from the IFC.
I have constructed the class in operator.py as following:
class ImageCollectionActions(bpy.types.Operator):
bl_idname = "image.collection_actions"
bl_label = "Execute"
action: bpy.props.EnumProperty(items=(("add",) * 3,("remove",) * 3,),)
index: bpy.props.IntProperty(default=-1)
def execute(self, context):
image_collection = context.scene.image_collection
if self.action == "add":
image_item = image_collection.items.add()
if self.action == "remove":
image_collection.items.remove(self.index)
#for item in image_collection.items:
return {"FINISHED"}
Now I have another class AddReferenceImage in which I want to get the path of the image stored .
I wasn't able to test right now, but would it work if you just add an index to th AddReferenceImage operator as well? And then the UI would be like:
for i, item in enumerate(image_collection.items):
row = box.row(align=True)
row.prop(item, "image")
op = row.operator("add.referenceimage", text="", icon="RIGHTARROW")
op.index = i
row.operator("store.referenceimage", text="", icon="PLUS")
op = row.operator("image.collection_actions", text="", icon="REMOVE")
op.action = "remove"
op.index = i
And how would I run unit and ui tests in python for a blender add-on? Also how to build a blender add-on to create a zip in github actions? Can't really find coherent documenation on these issues.
Comments
The length should be readonly, but when the user adjust the
N
orCenter to Center
it should see the updated length immedetialy.I asked chatGPT, but this code is missing something because it didn't do anything:
You have to initialize the callback on
my_n
andmy_center_to_center_distance
, notmy_length
:This should work ? BTW I don't think you have to tag the UI for a redraw here, the property changing should cause the UI to redraw.
Thank you, it worked.
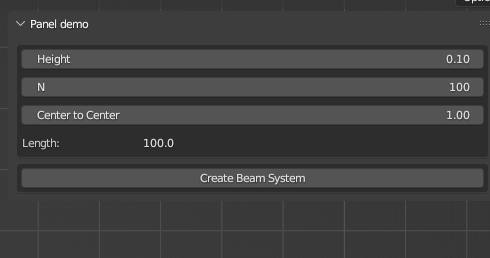
in
properties.py
in
ui.py
I have no idea what you mean by this ?
Hehe glad it worked :)
I mean you're using a
StringProperty
to hold the value ofmy_length
. It's representing a numerical value so I assume you have a reason to use a String Property instead of aFloatProperty
. There are valid reasons to do so, mainly because float values are limited in precision, as you can see in your gif when your change a value the last digits of the length kind of go haywire because of that. Strings can hold an infinite precision (at least in the needed significance range for construction projects).Internally it is stored as a string of characters, not as a numerical value. Python is a dynamic language which lets you do a lot of things without worrying too much about the underlying data structure, but you have to be careful about some things.
print("2")
andprint(2)
will yield the same result, howeverprint("2" + 2)
will raise an error. The interpreter can't assume how you want to compute non-trivial operations. So I'd recommend you to cast the value to the intended data structure when you're dealing with dynamic data types.print("2" + str(2))
will yield"22"
whenprint(float("2") + 2)
will yield"4.0"
Thanks for the help, I am trying to make some experimental add-on in which all the geometry is completed IFC based. Don't have any use case but I'm just exploring possibilities of what is possible and could be practical.
I hope this is a very simple question, but gooling gave me unsatisfying results:
I have the following add-on :
The workflow should be as following:
0) folder icon sets a path
1) > icon adds an image in Blender, so you could make transformations to the image in Blender, e.g. scaling, rotation and position
2) + icon saves the path images with transformation as an
IfcPropertySet
underIfcProject
3) Load image(s) from IFC loads the images with transformations from the IFC.
I have constructed the class in
operator.py
as following:Now I have another class
AddReferenceImage
in which I want to get the path of the image stored .the
ui
looks like this:How can I know in
AddReferenceImage
class whichindex
the user is clicking?Is the user clicking on the 0, 1, 2 3, etc. item of the collection?
I wasn't able to test right now, but would it work if you just add an index to th
AddReferenceImage
operator as well? And then the UI would be like:@bruno_perdigao
Yes thank you!
Now I can do in operator.py this:
How would I create environments in Python Blender without python defaulting to the system installed modules?
And how would I run unit and ui tests in python for a blender add-on? Also how to build a blender add-on to create a zip in github actions? Can't really find coherent documenation on these issues.
Hehe that's unfortunately above my skills. :)